Please note, the following article is targeted toward developers, designers, and webmasters comfortable with writing basic custom PHP. Making a mistake when writing PHP can cause fatal errors that may bring your site down until you can resolve the coding error. Ensure that you have a backup of your WordPress website before proceeding.
One of the coolest features of the BoldGrid Theme Framework and our WordPress website builder is the ability to “automagically” create a menu featuring your social media profiles, represented by their brand icons, quickly and easily through the WordPress-native menu feature. This is accomplished by creating a menu with “Custom Link” items, with URL’s linking to the most popular social media platforms.
The built-in Social Media platforms include the big ones, such as Facebook, Twitter, Instagram, and Pinterest. It also includes niche platforms like StackExchange, Yelp, and GitHub. However, some advanced users may want to add additional icons that are not included in the default icon set.
Add Code Snippet
This requires creating a WordPress filter with the value specifying the URL to search for in the Custom Link, as well as the Font Awesome code for the icon you wish to replace the text with. You can do this in a child theme’s functions.php file, or by using the popular Code Snippets plugin. These steps will assume you’re using Code Snippets, but the same code can be used in your functions.php file if you’re creating a child theme.
- If you haven’t already, install and activate the Code Snippets plugin.
- Navigate to Snippets -> Add New.
- Paste the following code, and customize it as needed: (don’t save it yet)
function bgsc_add_custom_social_network_imdb( $networks ) { $networks['imdb.com'] = array( 'name' => 'IMDB', 'class' => 'imdb', 'icon' => 'fa fa-imdb', 'icon-sign' => 'fa fa-imdb-square', 'icon-square-open' => 'fa fa-imdb fa-stack-1x', 'icon-square' => 'fa fa-imdb fa-stack-1x', 'icon-circle-open-thin' => 'fa fa-imdb fa-stack-1x', 'icon-circle-open' => 'fa fa-imdb fa-stack-1x', 'icon-circle' => 'fa fa-imdb fa-stack-1x', ); return $networks; } add_filter( 'boldgrid_social_icon_networks', 'bgsc_add_custom_social_network_imdb' );
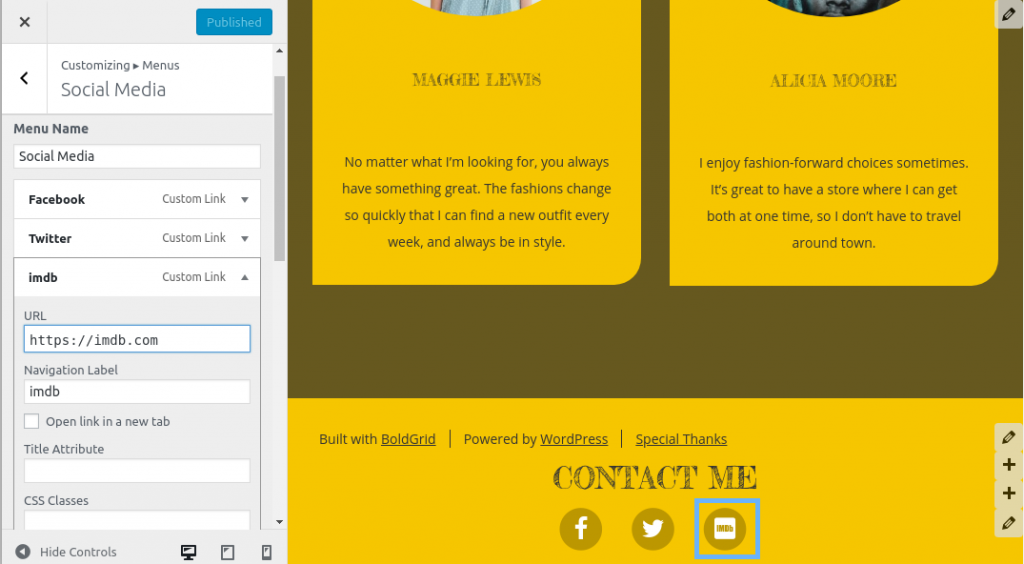
Code Explanation
Let’s break down this code line-by-line.
function bgsc_add_custom_social_network_imdb( $networks ) {
This is the function declaration for the filter callback function. This should be a unique function name, so it’s a good idea to prefix it with something unique to your website. In this example, we used bgsc_ for BoldGrid Support Center.
$networks['imdb.com'] = array(
This first part of line 2 takes the $networks array that was passed from the BoldGrid Theme Framework’s includes/class-boldgrid-framework-social-media-icons.php and adds a new entry to that array. The name of the element of the array, in this case, imdb.com, will also serve as the search term when the framework is replacing link text with icons. So in this case, any Custom Link menu item with imdb.com in the URL will be replaced with the custom social media icon.
'name' => 'IMDB', 'class' => 'imdb', 'icon' => 'fa fa-imdb', 'icon-sign' => 'fa fa-imdb-square', 'icon-square-open' => 'fa fa-imdb fa-stack-1x', 'icon-square' => 'fa fa-imdb fa-stack-1x', 'icon-circle-open-thin' => 'fa fa-imdb fa-stack-1x', 'icon-circle-open' => 'fa fa-imdb fa-stack-1x', 'icon-circle' => 'fa fa-imdb fa-stack-1x', );
This second part of line 2 is where you define the CSS classes and other properties for the icon. The name field will display when users hover over the icon, so make sure to specify that, in case your visitors are not familiar with the icon. Every Font Awesome icon must have the class fa, and then fa-(iconname). Some additional classes are added, depending on its specific use-case. For most purposes, you only need to worry about replacing fa-imdb with fa-(iconname). A full list of icon names can be found on the Font Awesome Cheat Sheet.
return $networks;
As the last part of the function, return the new array back to the BoldGrid Theme Framework, with your new item.
add_filter( 'boldgrid_social_icon_networks', 'bgsc_add_custom_social_network_imdb' );
Finally, use the WordPress add_filter() function to register your new function using the boldgrid_social_icon_networks hook provided by the BoldGrid Theme Framework.
Use Only Run Once for Safety
Before you save your code snippet, check the option for Only run once. If your website does not crash, you can safely check Run snippet everywhere and save again. This will prevent you from needing to enable Code Snippets Safe Mode to recover your website.
Adding an Envelope Email Icon
Here’s one more example that might be useful. By default, links with mailto: are replaced by the Envelope icon. However, if you don’t want your email address publicly available, you might instead opt to use another page on your website with a contact form that your users can fill out. You can use the page slug of your contact page as a search term, and replace that link with the same envelope icon:
function bgsc_add_custom_social_network_email_form( $networks ) { $networks['/contact-us/'] = array( 'name' => 'Contact Us', 'class' => 'envelope', 'icon' => 'fa fa-envelope', 'icon-sign' => 'fa fa-envelope-o', 'icon-square-open' => 'fa fa-envelope fa-stack-1x', 'icon-square' => 'fa fa-envelope fa-stack-1x', 'icon-circle-open-thin' => 'fa fa-envelope fa-stack-1x', 'icon-circle-open' => 'fa fa-envelope fa-stack-1x', 'icon-circle' => 'fa fa-envelope fa-stack-1x', ); return $networks; } add_filter( 'boldgrid_social_icon_networks', 'bgsc_add_custom_social_network_email_form' );
This example presumes that your contact form page URL contains the string /contact-us/, and then replaces links to that page with the custom envelope icon.
Add the TikTok Icon
Here’s another example for adding the TikTok network to your social media menu. Please note that you will also need the official Font Awesome plugin to get the newest set of icons.
function bgsc_add_custom_social_network_tiktok( $networks ) { $networks['tiktok.com'] = array( 'name' => 'TikTok', 'class' => 'tiktok', 'icon' => 'fab fa-tiktok', 'icon-sign' => 'fab fa-tiktok-square', 'icon-square-open' => 'fab fa-tiktok fa-stack-1x', 'icon-square' => 'fab fa-tiktok fa-stack-1x', 'icon-circle-open-thin' => 'fab fa-tiktok fa-stack-1x', 'icon-circle-open' => 'fab fa-tiktok fa-stack-1x', 'icon-circle' => 'fab fa-tiktok fa-stack-1x', ); return $networks; } add_filter( 'boldgrid_social_icon_networks', 'bgsc_add_custom_social_network_tiktok' );
Congratulations! Now you know how to add custom social media icons to your BoldGrid website.
SIGNUP FOR
BOLDGRID CENTRAL
200+ Design Templates + 1 Kick-ass SuperTheme
6 WordPress Plugins + 2 Essential Services
Everything you need to build and manage WordPress websites in one Central place.
cindy says:
thank you!!!!!
Arthur says:
Is there a way to download chosen Icons placed on my site so that my print materials are consistent?
Brandon says:
Hi Arthur!
The “Icons” you see in Post and Page builder are all apart of the Font Awesome icon library. The best way to obtain them is to download then directly from Font Awesome. This way you’ll have all access to all downloadable file types CSS syntax.
Travis says:
Is it possible to use a square/rectangular PNG or SVG file as the icon instead of a Font Awesome glyph?
Jesse says:
Hi Travis-
Since the icons are inserted with CSS, it should be possible to create some custom CSS to use your custom SVG, but this would take some specialized development to accomplish.